Pre-requisite:
Ensure the basic integration of the Nudge Core SDK is complete. If not, check here.
Overview
The nudge_flutter_stories
package lets you add interactive stories to your app. It includes the NudgeStories and NudgeStoriesUi widgets for displaying and customizing stories. Install this plugin to launch stories from the dashboard and increase user engagement.
Installation
Add nudge_flutter_stories
to your pubspec.yaml
file:
dependencies:
nudge_flutter_stories: ^latest_version
Run the following command to fetch the package:
flutter pub get
Step-by-Step Guide
Step 1 : Import the required classes.
Import the NudgeStoriesUi
class and NudgeStories
widget from the nudge_flutter_stories package:
import 'package:nudge_flutter_stories/features/Stories/presentation/pages/stories_ui.dart';
import 'package:nudge_flutter_stories/nudge_stories.dart';
Step 2: Initialize and add to NudgeProvider
Create an instance of the NudgeStoriesUi
class :
final stories = NudgeStoriesUi();
Add this instance to the plugins
list in the NudgeProvider
return NudgeProvider(
nudgeInstance: core,
plugins : [stories]
child: MaterialApp(
navigatorKey: NudgeProviderState.navigatorKey,
navigatorObservers : [_trackerObserver],
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: Home(),
),
);
Step 3: Add NudgeStories Widget
Place the NudgeStories
widget in your app where you want to display stories, and call the track function for the event using the track
method provided by the nudge_core
package:
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
const NudgeStories(),
ElevatedButton(
onPressed: () async {
nudge.track(type: "TRIGGER_TYPE");
},
child: const Text(
'Get Stories',
style: TextStyle(fontSize: 20),
),
),
],
),
),
);
}
Example
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:nudge_core/nudge_core.dart';
import 'package:nudge_core/nudge_labels_manager/NudgeTrackerObserver.dart';
import 'package:nudge_core/nudge_provider.dart';
import 'package:nudge_flutter_stories/features/Stories/presentation/pages/stories_ui.dart';
import 'package:nudge_flutter_stories/nudge_stories.dart';
void main() {
runApp(
MainApp(),
);
}
String apiKey = 'API_KEY';
Nudge nudge = Nudge(
apiKey: apiKey,
);
String token = "UNINITIALIZED";
String nudgeId = "UNINITIALIZED";
class MainApp extends StatelessWidget {
MainApp({super.key});
final NudgeTrackerObserver _trackerObserver = NudgeTrackerObserver();
@override
Widget build(BuildContext context) {
SystemChrome.setPreferredOrientations([
DeviceOrientation.portraitUp,
]);
nudge.initSession(externalId: "EXTERNAL_ID");
final stories = NudgeStoriesUi();
return NudgeProvider(
nudgeInstance: nudge,
plugins: [
stories,
],
child: NudgeStoriesProvider(
child: MaterialApp(
theme: ThemeData(
primarySwatch: Colors.blue,
useMaterial3: true,
),
routes: {
"/": (context) => const HomeScreen(),
},
navigatorKey: NudgeProviderState.navigatorKey,
navigatorObservers: [_trackerObserver],
),
),
);
}
}
class HomeScreen extends StatefulWidget {
const HomeScreen({super.key});
@override
_HomeScreenState createState() => _HomeScreenState();
}
class _HomeScreenState extends State<HomeScreen> {
@override
void initState() {
super.initState();
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
const NudgeStories(),
ElevatedButton(
onPressed: () async {
nudge.track(type: "TRIGGER_TYPE");
},
child: const Text(
'Get Stories',
style: TextStyle(fontSize: 20),
),
),
],
),
),
);
}
}
Handling multiple scrollable widgets
Incase you plan to add our story widget along with an existing scrollable widget, you refer to the below snippet to handle the scroll across both widgets seamlessly.
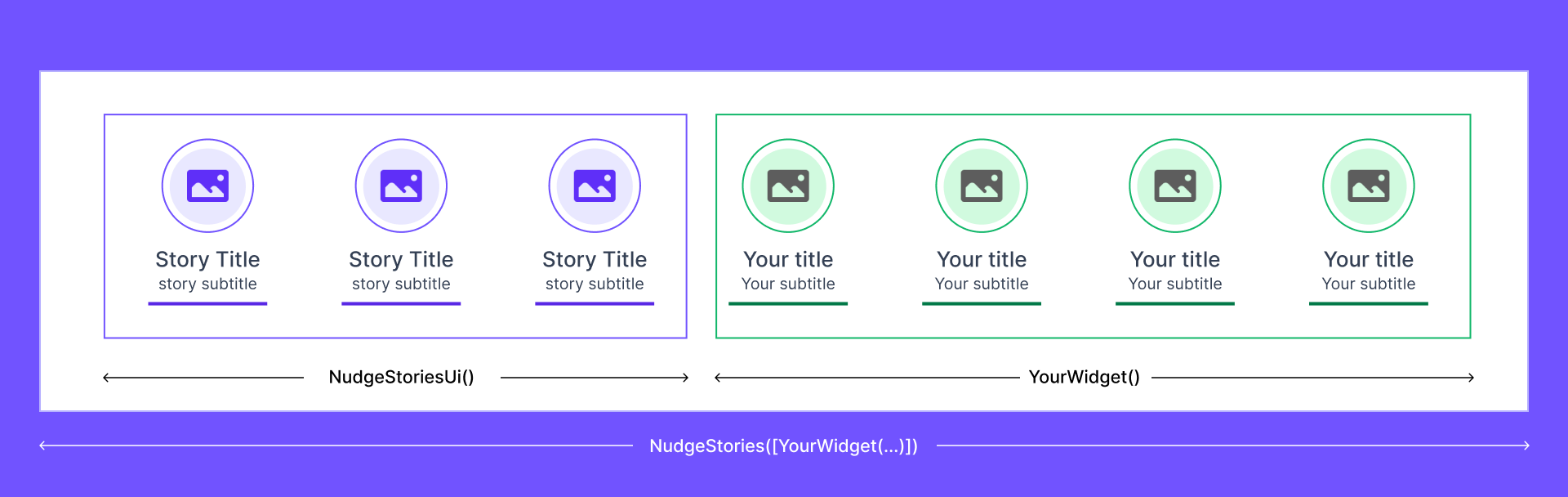
const NudgeStories(
customContent: [YourWidget1(...), YourWidget2(...), ...] // @type: List<Widget>
),